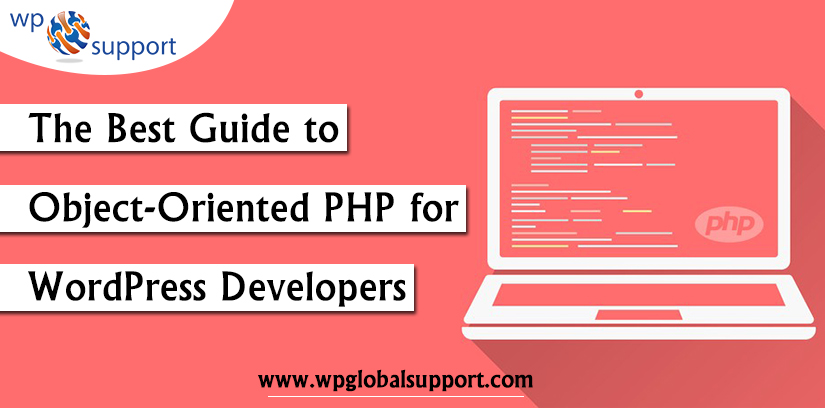
Are you looking for the best Guide to Object Oriented PHP for WordPress Developers? The first step in leveling up your skills as a developer is learning object-oriented programming for PHP or OOP.
OOP is all about more than using classes in your code. It’s about creating code that is less focused on a particular action. It is more focused on the object’s small reusable containers for functionality and data.
However, WordPress users don’t require to learn PHP for managing their WordPress-powered websites. You will need to have a basic understanding of PHP. If you’re a plugin or theme developer or just want to modify the default behavior of your Website.
This guide will help you with the knowledge and skills you need to get started with object-oriented PHP as a WordPress developer including the following things:-
- PHP fundamentals
- Object
- Instance
- WordPress’s Objects and much more.
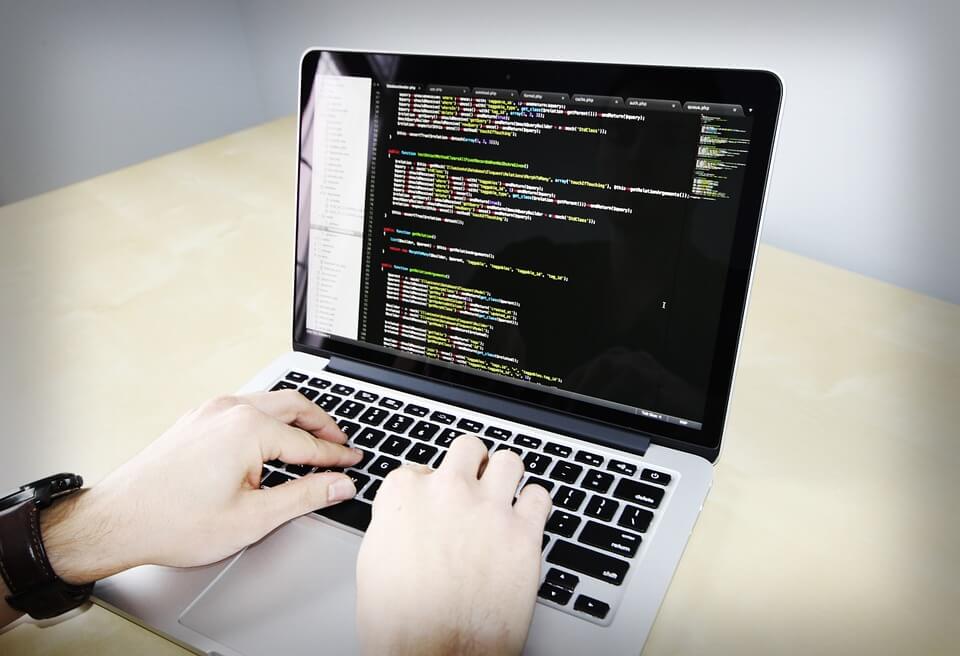
An Overview of Object
Generally, understanding object-oriented programming must start from an understanding of objects. The whole pattern builds from the realization that we humans relate to objects many times.
An object is a combination of properties and behaviors. Without thinking about it when entering a new room my brain reports things like “chair”, “person”, “cat”, “carpet”, “Table.” This is a miracle if you think about this from the level that our eyes, ears understand. Our brains casually do this and it’s one of the most profound speed bumps that we humans have had in trying to teach computers to think and work like us.
Humans have this great superpower of conceptualization. This is so remarkable we don’t even realize it exists. If you tried to layout the visual phenomena that let you classify some things as “table” and other things as “not table” you actually wouldn’t even know where to start. Is it about a size? Not actually. Color? Certainly Not. I could go on but will spare us both.
What Is Object-Oriented Programming?
OOP gives us the ability to group together a set of properties and behaviors into an entity or object.
Object-oriented programming often abbreviated as OOP to be a little less awkward mouthful builds on this superpower. The core thing that procedural code lacks that OOP gives us is the ability to group together a set of properties and behaviors into an entity or object that we find it a little simple to think about.
This lets us have a “User” object in a programming system as opposed to a unique identifier we require to remember comes from a specific “user account.” It lets us get more complex and define things like a “View Object” which has particular properties that we’re able to think about and expect across a variety of project technologies and languages.
What is the Instance?
While using the new keyword in PHP we’re “instantiating” the object. We create a new instance of it.
Instances can have specific ways in which they change but like dogs, they usually behave in similar ways. All dogs bark but their volume varies. And all dogs have fur but the color and length vary. All dogs can run and walk but the specific speed of each varies.
So Robin Q is a black dog with 1cm long hair who runs at 10km/hr. And Sam is a brown-and-white dog with 3cm long hair, who runs at 8km/hr. Both dogs can run, walk, or bark.
Properties & Methods
As I have said in the last paragraph that Robin Q is black. The $color property for the Dog instance named Robin Q is black. Likewise, the $color property of Sam is brown and white. Same with these dogs these properties of objects can sometimes change over their lifetime. Many of the dogs get slower in old age and that’d be reflected in their speed property decrementing over time.
Likewise, dogs can bark we think of this as a method on the object. Methods do not change between different instances of a class. Unlike properties nor do they change over the life cycle of an object.
Property is a fancy name for an object’s internal variables. In all cases, properties and methods are similar to what you’ve seen before with PHP functions and variables but with one vital difference.
The $this keyword, and arrows
While referring to an object’s properties or methods you’ll always use an arrow (->) in PHP. So in case, I have a $dog instance floating around, I call its bark method as $dog->bark().
If I want to reach its color I find that at $dog->color. This is what makes a distinction between properties and methods from variables and functions. Object are bags which hold our variables and functions together into more understandable and useful units.
You say something like if you’re outside of an object $object->method(). If you’re inside of the object say in its freak_out method, how to access its bark method? That’s where the $this keyword or magic variable comes in PHP.
In case I’m inside of my Dog classes’ bark method, and I wish to refer to my dog’s volume property, I do that with $this->volume. You’ll observe a slightly disturbing difference in PHP here: property declarations have a $ before them, $volume. In use either inside or outside an object, they don’t.
Privacy (Private vs Protected vs Public)
Sometime you’ll want your methods and properties to only be accessible by you on the inside of your class $this calls but not $object ones. You’d do this for reasons like methods that are helpful for what you want to do publicly and don’t wish to repeat but which an outsider would never have the use for.
For doing this in PHP, you’d declare the property private when you declare it. That looks like:
class MyClass {
private $property; } |
It’s not needed in PHP but useful and highly suggested to explicitly declare a class’s properties at the top of it. PHP still works if you don’t. And if you don’t declare whether a property is private it defaults to public.
Both properties and methods can be public or private. In both of the cases, that keyword just goes before the line declaring it. There is, as we’ll detail in a bit a third privacy status in PHP protected. We’ll illustrate what’s that is for under the “Subclasses” section below where it makes a little more sense.
Related Article: How to turn off PHP errors in WordPress Website?
Pause — Let’s See All of That in Code
So far I’ve run through all this stuff without much code that shows off the ideas. I want to take a second to do that so you have some sense of how all of this hangs together when you write it. So this has most of the important details we’ve discussed before:
class Dog {
public $color; private $volume; public function bark() { text_to_speech(“arf”, $this->volume); } } $fido = new Dog; $fido->color = ‘brown’; echo $fido->color; $fido->bark(); |
Inline one we declare our class. You’ll see that the class name starts with a capital letter this is customary to better tell between classes and functions but is not needed. It is also customary in the WordPress ecosystem for separating multiple-word class names with underscores. My_ManyWord_Class is less common outside of WordPress whereas CapWords without the underscores is more common.
On the next two lines, we reveal the properties color and volume marking them public and private. At last, we define a public bark method that uses a theoretical text_to_speech function you’ll see that in neither PHP or WordPress which uses that volume property as the second argument.
That ends the class definition. Then we make an instance of our Dog class, Sam. We set Sam’s color to brown and then echo that value. After that, we call the public bark() method on the $fido object again an instance of our Dog class.
What & Why of Subclasses
The other thing that matters for WordPress most clearly for widgets is called “subclassing” or “object extension.” These apparently opposed terms mean the same thing and they point to when you borrow some of the properties and behaviors of a parent object but override some others.
Given our Dog class, we can think of something like Raka as a subclass. For say a height property rather than a default of 10 arbitrary units, we’d expect a Raka to have something like 5. That would look like.
class Dog {
public $height = 10; } class Raka extends Dog { public $height = 5; } |
In this case, the bark method (unshown) of Dog is automatically found on the Raka class as well because that exclass “extended” the Dog one. We’d also automatically get other not shown here default properties, magic methods, etc. Any of the things you don’t explicitly override when you extend or subclass is automatically the same on that subclass.
See Also: WordPress Memory Exhausted Error – Increase PHP Memory limit
WordPress’s Objects
There are a few places that you’ll likely encounter objects in PHP. This first is WP_Query. WP_Query is a helpful way you can get a new set of posts that is any content type, like pages, posts, etc from the database.
Another way you can’t really avoid it is if you’re making WordPress widgets. When making widgets you subclass (extends a WP_Widget class), overriding specific methods.
It’s the case that many things WordPress functions return are objects under the hood. The $post global that the_title access is an object. You can also search for the user objects and more as query results. You’ll mostly just want to understand property access like $user->ID as you’ll not actually be writing or overriding those objects yourself in most of the cases.
Plugin for one object?
The other place you will see the WordPress developers apply OOP frequently is in plugin development.
Generally, you’ll see plugins either managing many objects or just making one single object which contains all the plugin’s behavior. There are good and bad reasons that one should use an object or many objects when developing a WordPress plugin.
For doing good OOP done perfectly is a great thing. To the bad, I think OOP did because you think it’s the correct way and doesn’t understand how it’s meant to help adds confusion and little value.
You should probably define one or many objects if you have a WordPress plugin of more than ~100 lines of code. But it’s necessary to think critically about what those objects are and do.
Using WordPress Hooks with Objects
One common pattern inside of OO PHP in WordPress plugins that I find irritating, however, is an over-WordPress constructor. WordPress plugins need the use of hooks, actions, and filters for interacting with WordPress. Putting them in the constructor of your object chains your object to WordPress. Making it hard to override and tests on isolation the things it does.
So it is better to expose an object’s public methods and have a bit of imperative or procedural code outside of the object that hooks them in.
OOP and WordPress – The Best Match
WordPress is a very old software project as it is very young relative to human history. As such a lot of it was written by people who weren’t fully sold on object-oriented PHP or before OOP in PHP became the de facto standard. As such, many codes in WordPress is not very object-oriented.
Conclusion
It’s helpful even if you write basic procedural functions and not objects to understand what the object-oriented code you will encounter literally does. I hope this has been useful for you to learn oop in WP.
That’s all on our behalf and we hope that you read this blog about Object-Oriented PHP thoroughly.
In case of any help related to WordPress Backup and Recovery feel free to contact our WordPress Backup and Restore team, dial +1-888-738-0846(Toll-Free).
That’s all, thanks!