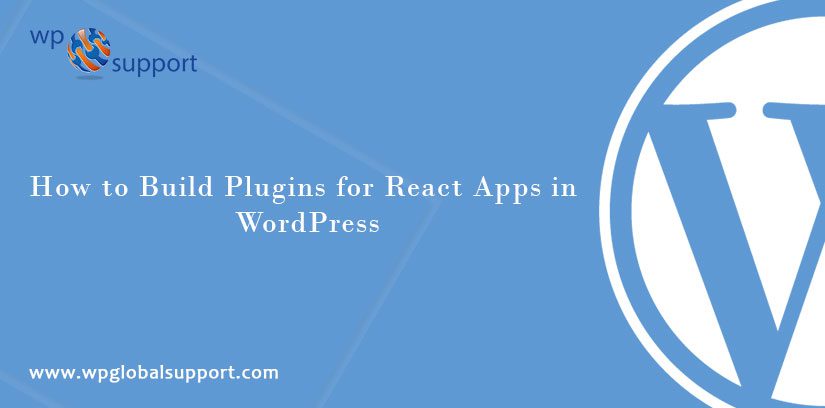
Do you want to build Plugins for React Apps in WordPress? WordPress is a powerful content management system that has been widely used for creating websites and blogs. In recent years, React has gained a lot of popularity among web developers for its ease of use and flexibility. Combining the power of WordPress and React can lead to the creation of highly functional and responsive websites.
In case you’re not creating an app that needs plugin support, you can then also apply these concepts to create more powerful large React apps. In this article, we will show you how to build a plugin for React Apps in WordPress.
What is React.js?
React JS is a JavaScript framework that has been under development by Facebook since 2012. The main cause that you are hearing more about it recently is because of the fact that Facebook did not publicly announce the project until 2015.
However, thousands of PHP developers had to become articulate with JavaScript to be able to take benefit of the new tech stack and create apps on top of WordPress the React way.
Read Also: Adding Javascript and Styles in WordPress
Advantages of Using React App
- React isn’t a framework as it is a library that gives an informative method of defining UI components.
- Access to the native world. React App Native allows you to create mobile apps using only JavaScript.
- Complete separation of data and presentation. React App provides slightly more than a presentation layer.
- Writing React template is very similar to writing HTML with interpolation thanks to JSX.
Disadvantages of Using React App
- Build tools are important for all front-end apps but the simplest, decent build tools are highly suggested.
- Restrictive Licensing
Why Plugins are Required?
There are many plugins for customizing the authoring interface, adding a brand new sidebar, registering their own settings panels, and much more. It’s very powerful as the WordPress dashboard supports plugins. You can also create a mobile app with WordPress.
Where plugin is defined as a type of software that contains a group of functions that can be added to a WordPress website. They are used to provide additional functionality to your application.
As Sidebar is a theme feature, which is basically a vertical column provided by a theme for displaying information other than the main content of the web page. The usage of sidebars changes and it depends on the choices of the theme designer.
Also, there is a group of stylesheets and templates that are used to describe the appearance and display of a WordPress site. And, templates are those files that control how your WordPress site will be displayed on the Web. WordPress is written in PHP and plugins are completely rendered on the server.
That was brilliant back in 2004, but more than a decade later, things have changed. If rebuilt today, the WordPress dashboard would possibly be a client-side JavaScript application written in React, and it would support plugins as first-class objects.
Read More: List of Top 10 WordPress Plugins To detect Malicious Code in your site
Supporting Plugins With React App
React App grants you illustration self-contained components so it’s the best foundation for plugins. But once we started creating, we found three major key issues:
- Plugin components require to access the state and want to implement features that differ from the state in uncertain ways.
- Plugin components need to display somewhere in the application, but other components don’t know how to render them. Adding them straight to the DOM will produce invariant errors.
- They may throw exceptions, and they could crash the whole app.
Extending the Flux Pattern
We are extending the Flux Pattern for Shared States in Large Applications. Letting unknown 3rd party components mutate shared states becomes largely problematic in large apps. And We’ve fixed this by integrating our Flux stores and exposing flexible interfaces for components.
What is Flux?
For a complete understanding of Let’s see the example of building a simple chat app, having a list of messages in its main view. This is the way how state flow works via the Flux pattern.
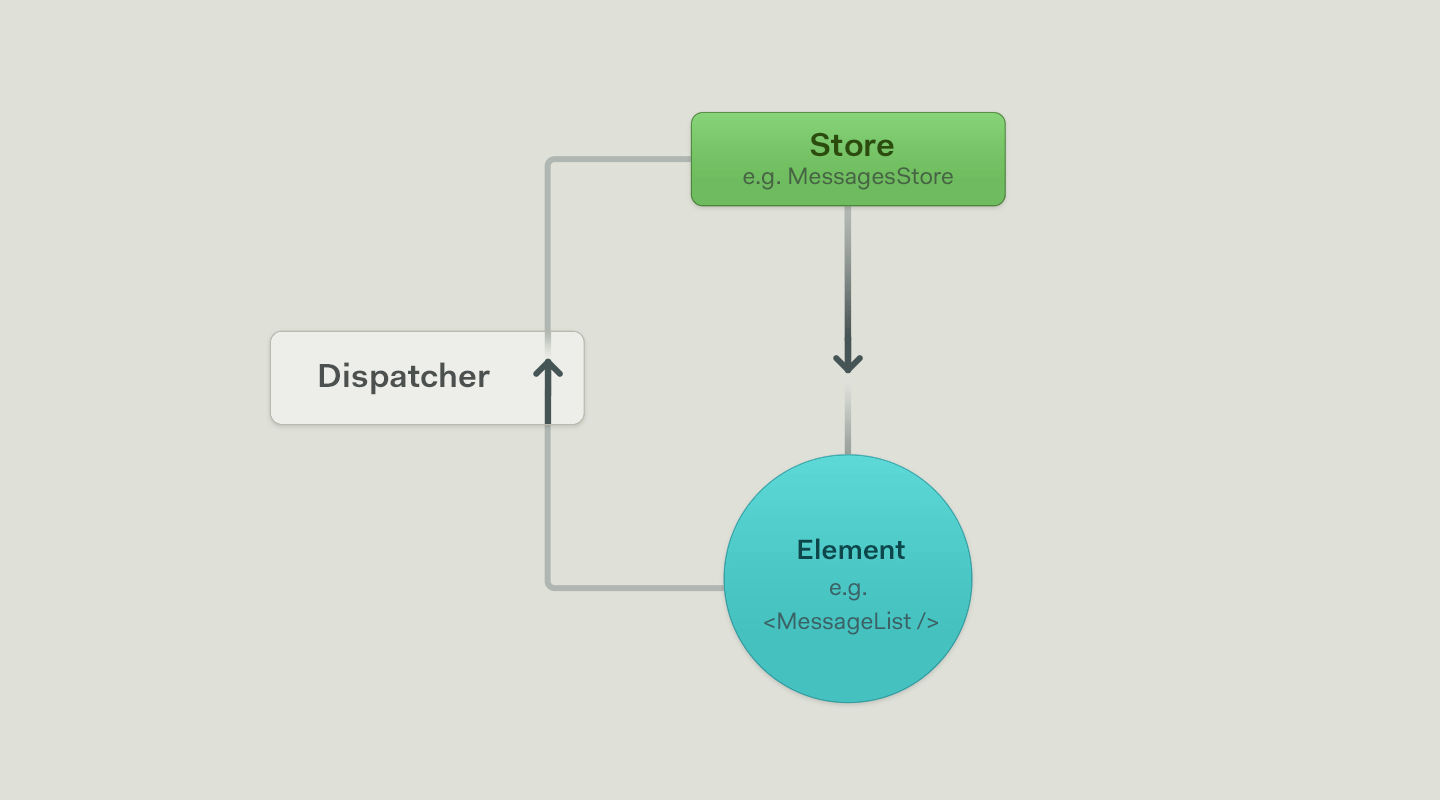
- A green Store is an object that holds data or information. In the above example, it is holding a list of messages so we call it MessageStore.
- The blue Component is liable for rendering data. In the above example, this renders the list of messages.
- The global dispatcher controls routing actions and events generally from a component back to a store. Clicking on a “star” button on a message will broadcast an action all over the system, which would update the store and cause components to re-render. Flux makes it very difficult for views to get out of sync with the underlying state.
From One Store to Many
If you need to extend this chat app for managing multiple ongoing threads with various people. This is very easy as we just create a ThreadStore and ThreadList. At the time a new thread is chosen, the MessageStore requires to change the list of active messages in order for the MessageList to re-render. We do this by having the MessageStore listen to events from the ThreadStore.
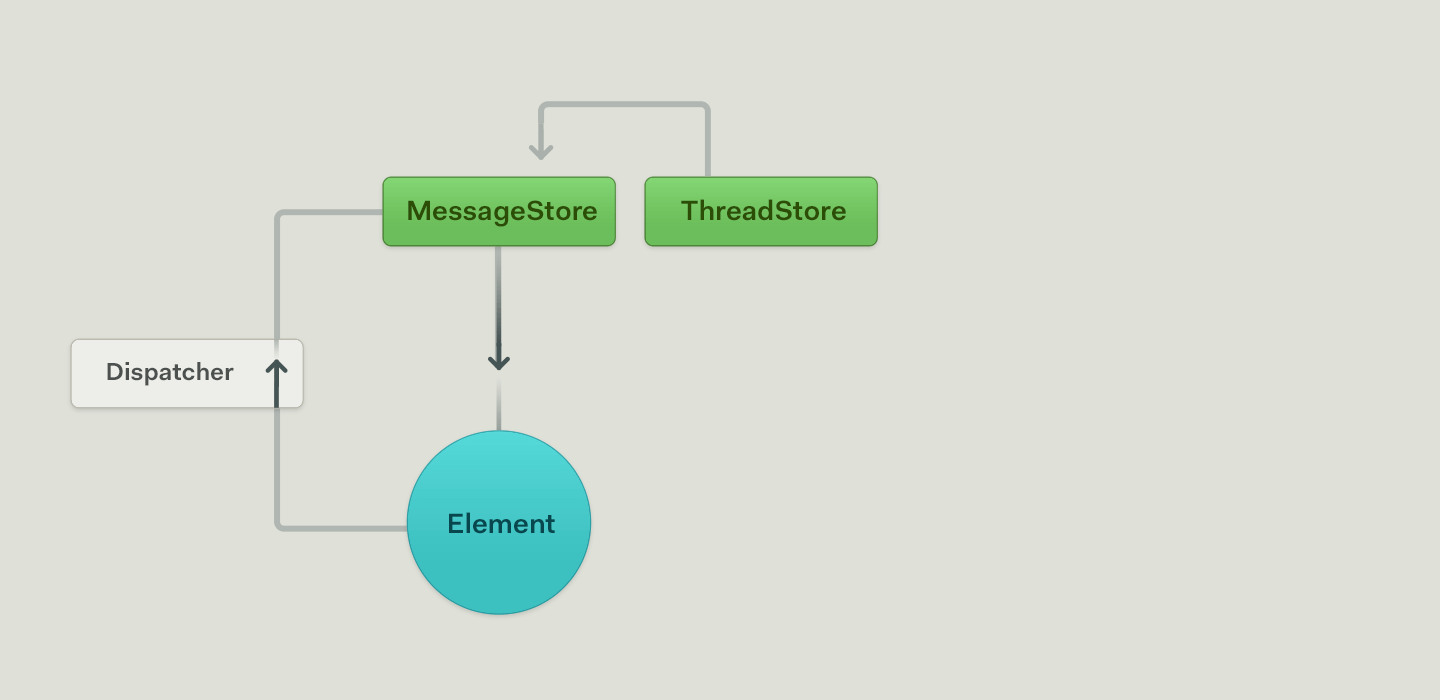
Linking stores are also a usual design pattern in Flux, and provide a way to interdependent data structures. Adding more connected stores can make this fastly grow out of control. It’s difficult to reason about where data is being changed completely, and easy to announce complicated circular dependencies. This doesn’t scale, specifically with plugins.
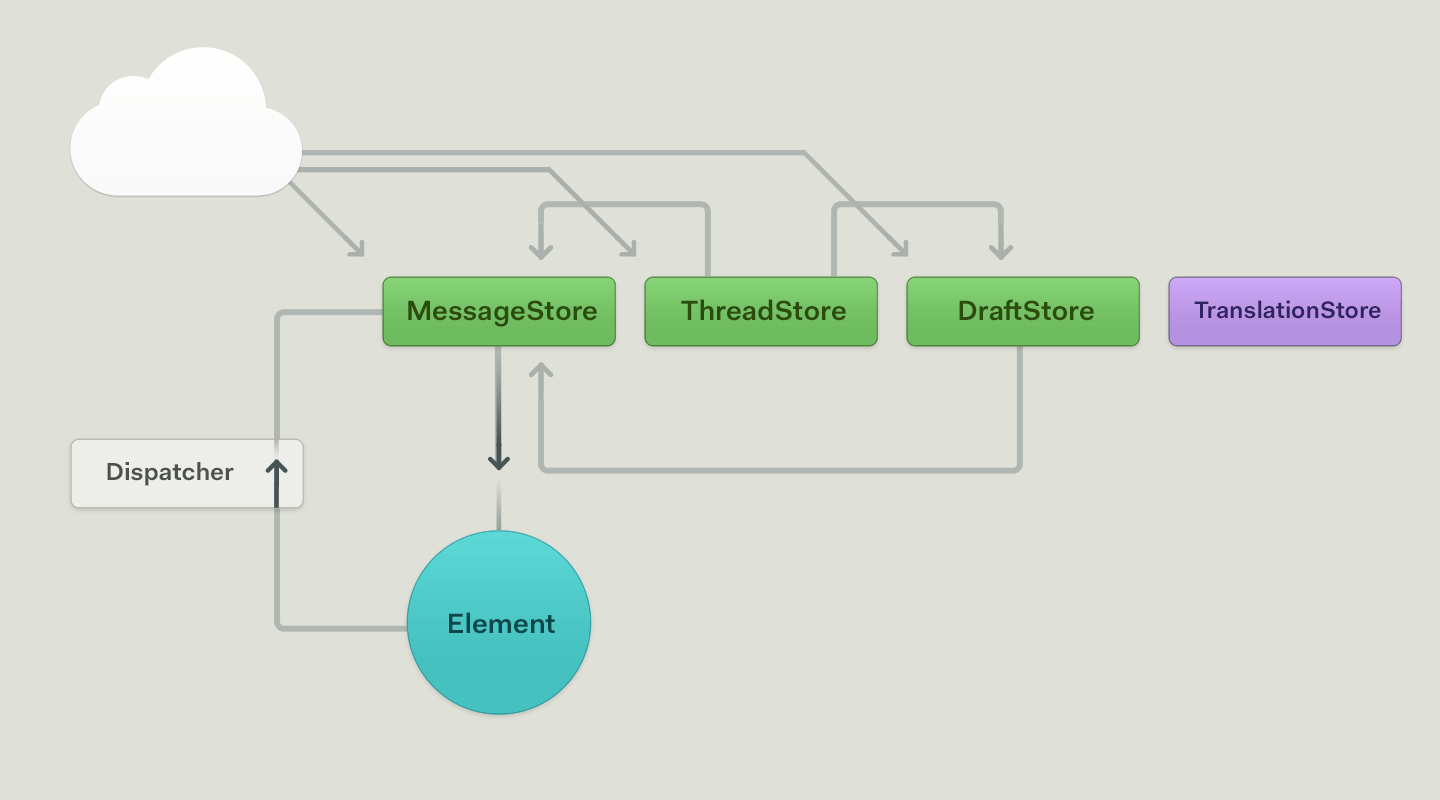
For example, if you need to create a translation plugin. This plugin generally needs to transform the data, so we have to write a TranslationStore as shown in purple. It will translate outgoing messages from the DraftStore and also publish translated messages to the MessageStore.
The OmniStore
Our quick solution is to centralize the app’s data in an OmniStore. It is a single top-level store that acts as a source of truth and removes the dependency chain. This store is just like a frequent Flux store, except that it now holds all the primary application states. Via this design, other stores only cache and aggregate subsets of the central data, and sell it to individual components.
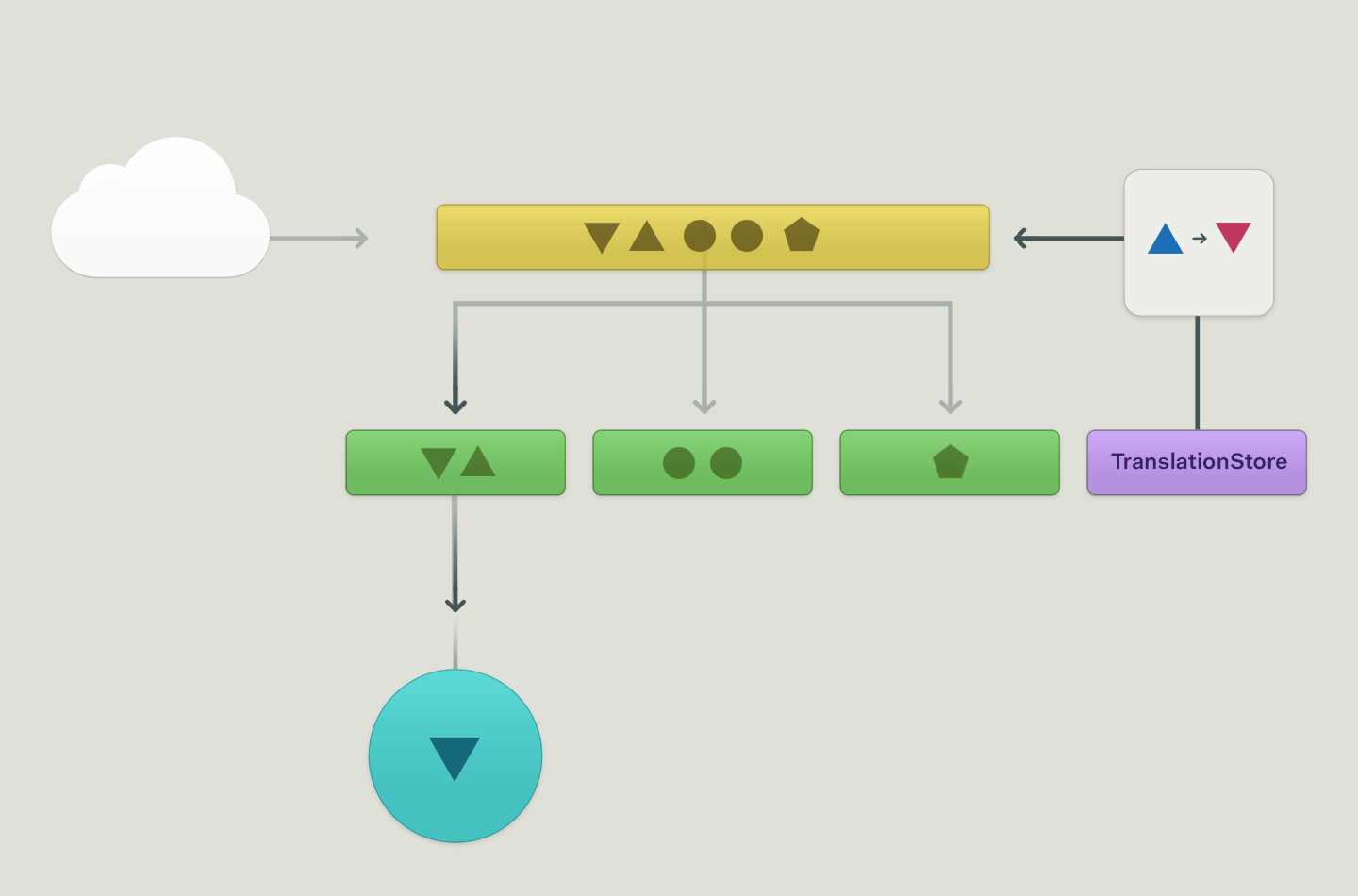
This pattern is simpler to reason about when creating and debugging. Decoupling earlier dependent stores grants us to safely introduce 3rd party plugin stores. Now the Translation Store can straightly update data in the Omni Store (yellow), which will broadcast notifications and occur the significant components to re-render.
Dynamically Injecting React Components
We introduced a 3rd party plugin for translating messages in the previous example. But this plugin possibly also requires a “translate” button at the bottom of the message composer next to the send button. Here’s what the JSX might look like:
static-components.jsx <div id=“actions”> <UndoButton draft={this.props.draft} /> <AttachFileButton draft={this.props.draft} /> <SendButton draft={this.props.draft} /> // new component here! </div> |
The current draft is passed as a prop to every one of the three buttons. But for enabling our React app to dynamically inject a new TranslateButton:-
- First of all, move the list of buttons we are rendering from JSX to an array. This is a small React trick and will render the accurate same content. Our app can easily pass the same props to all of them as each button’s styling and behavior is self-contained. Whereas an array uses just one variable and lets you save multiple pieces of data using the same variable.
component-variables.jsx
render: function() { var componentClasses = [ UndoButton, RedoButton, AttachFileButton ]; return <div id=“actions”>{ componentClasses.map(function(componentClass){ return <componentClass draft={this.props.draft}/>; }); }</div>; } |
- Next upgrade the components array into a Flux store, This new Component Store has a componentClasses() method to return an array of component constructors. The new toolbar component requires noticing the Component Store and rendering the passed component items.
injected-components.jsx
<div id=“actions”> <InjectedComponentSet location={‘composer-actions’} passedProps={ {draft: this.props.draft} } /> </div> |
Our major goal is to write functions that perform on data and return data. JSX is a syntactic sugar for the usual data shape we use all the time in React. And if we treat views as just data, we can take benefit of the same Flux patterns used throughout the app.
Maintaining an index of components in a Flux Store has a few benefits. Components can leverage actual mixins and patterns, and listen to the store for changes in available components. This permits the app to stay in sync as plugins are loaded and unloaded. We can also build Flux Actions for registering and unregistering components, which makes these processes globally viewable within the application.
The props passed to an injected component are necessarily the component’s API. They can be obviously documented for developers, and display the true application state (unrelated to the DOM state).
Isolating Unknown Components
One of the drawbacks of a globally reactive application is that exceptions in the lifecycle or render methods can sink the whole app.
And we fix this by rendering components in a new React root. That means we render a placeholder div, and then manually call React. render within a try/catch block. For managing the lifecycle, we trigger this on all component update events (componentDidUpdate / componentDidMount) and also call
React.unmountComponentAtNode when components unmount.
React. render is created to be flexible, and supports rendering fresh or updated existing views.
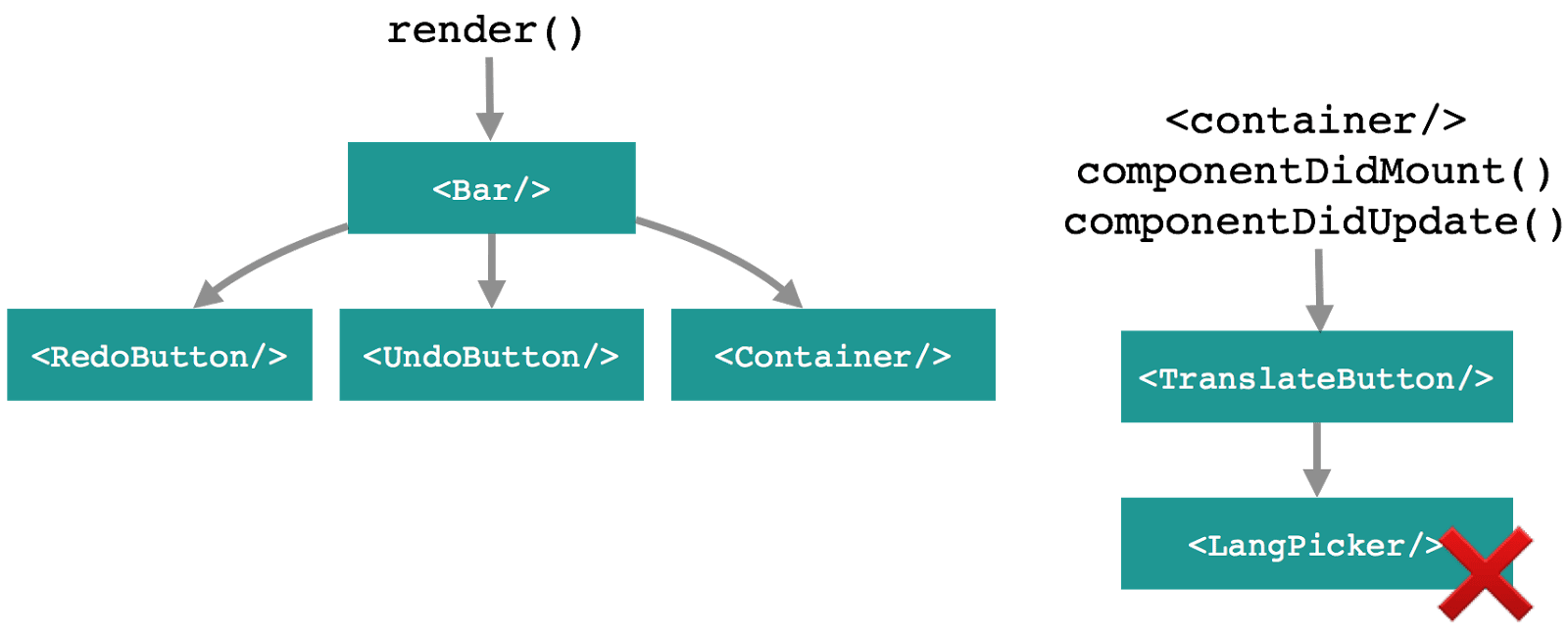
This lets us handle any errors in the render methods, and securely isolate, kill, and report poor-quality components. We can display helpful error messages to users and developers when things go wrong.
CLOSING THOUGHTS
We hope that this article helped you in building Plugins for React Apps. As you can easily build it by reading the above article carefully.
You can contact to our WordPress Customer Service team just dial +1-888-738-0846(Toll-Free) for the instant help and service relate to WordPress.