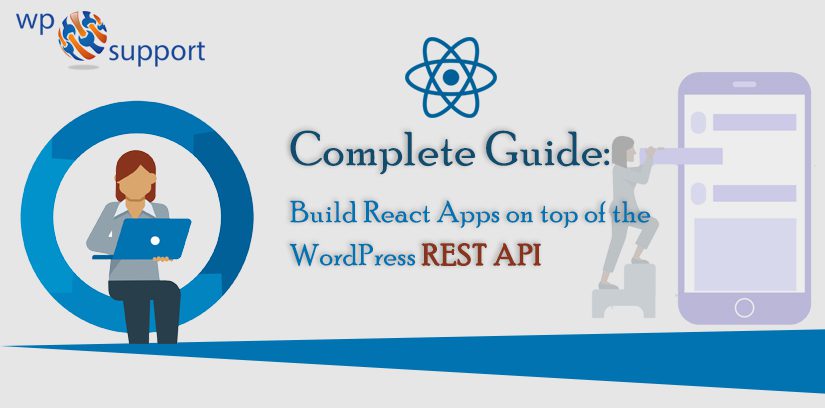
Learn how to Build React Apps on top of the WordPress REST API. WordPress is a most popular and powerful CMS (Content Management System). But when it comes to developing on top of it is quite frustrating. The crazy mixture of WordPress of HTML and PHP loops can often prove to be rude in understanding and maintaining unimaginable.
In this blog, we are going to use ReactJS to build the ReactJS for development app front end, React Router for routing and webpack to keep it together. Also, we will show you how to integrate advanced custom fields too to create a very intuitive solution.
Let’s get started!
Project Setup
Suppose the name of the project “wp-api-react”. To follow along, whatever you have to do, it is included in your package.json and run npm install:
{
“name”: “wp-api”, “version”: “1.0.0”, “description”: “”, “main”: “index.js”, “dependencies”: { “alt”: “^0.18.6”, “axios”: “^0.15.3”, “lodash”: “^4.17.4”, “react”: “^15.4.2”, “react-dom”: “^15.4.2”, “react-router”: “^3.0.1” }, “devDependencies”: { “babel-core”: “^6.21.0”, “babel-loader”: “^6.2.10”, “babel-preset-es2015”: “^6.18.0”, “babel-preset-react”: “^6.16.0”, “react-hot-loader”: “^1.3.1”, “webpack”: “^1.14.0”, “webpack-dev-server”: “^1.16.2” }, “scripts”: { “test”: “echo \”Error: no test specified\” && exit 1″ }, “author”: “”, “license”: “ISC” } |
Install WebPack and WebPack-Dev-Server globally, even if it has not been done beforehand.
npm i webpack webpack-dev-server -g
Now, create index.html and webpack.config.js in the project folder. The index.html file will contain a piece of code as shown below:
<!DOCTYPE html>
<html> <head> <title>WP API</title> </head> <body> <div id=”app”></div> <!– This div will be holding the app –> <script src=”bundle.js”></script> <!– This is the main JS file that gets bundled by webpack –> </body> </html> (BI) |
Coming back to webpack.config.js, A file makes the project apart from listening to live updates.
Paste the following code in webpack.config.js:
(BI)
var webpack = require(‘webpack’); var path = require(‘path’); module.exports = { devtool: ‘inline-source-map’, // This will show line numbers where errors are accured in the terminal devServer: { HistoryApiFallback: true, // It will create server understand “/some-link” routs instead of “/#/some-link” }, entry: [ ‘webpack-dev-server/client?http://127.0.0.1:8080/’, // Specify the local server port ‘webpack/hot/only-dev-server’, // Enable hot reloading ‘./src/index’ // This is where Webpack will be looking for the entry index.js file ], output: { path: path.join(__dirname, ‘public’), // It is used for specifying a folder for this productive bundle, will not be used here, but it is a good practice to do it. filename: ‘bundle.js’ // Filename for production bundle }, resolve: { modulesDirectories: [‘node_modules’, ‘src’], // Folders where Webpack is going to look for files to bundle together extensions: [”, ‘.js’] // Extensions that Webpack is going to expect }, module: { // Loaders allow you to preprocess files as you require() or “load” them. Loaders are like “tasks” in other build tools, and provide a powerful way to handle Frontend Build steps. loaders: [ { test: /\.jsx?$/, // Here we are going to use ReactJS for react components. But including JSX in case this extension is prefered exclude: /node_modules/, // Speaks for itself loaders: [‘react-hot’, ‘babel?presets[]=react,presets[]=es2015’] // Modules that help with hot reloading and ES6 transcription } ] }, plugins: [ new webpack.HotModuleReplacementPlugin(), // Hot reloading new webpack.NoErrorsPlugin() // WebPack will tell you if there are errors ] } |
And this in webpack.production.config.js:
It is recommended to add some more folders to the project, create a folder named “Src” and a folder “component” and further index.js will be made.
Therefore, the folder’s structure will look as below:
wp-api/
— node_modules/
— src/
— — components/
— — index.js
— index.html
— package.json
— webpack.config.js
There’s a lot of entry points for the index.js webpack which will also have all the routes for the project.
Dynamic Routes
Currently, only index routes have been set in the app. This will limit the chance to bring pages created on the front page on the WordPress backend.
We need to create a method in index.js to dynamically add routes to Reacts router. Give this method be
buildRoutes():
import React from ‘react’; import {render} from ‘react-dom’; import App from ‘./components/App.js’; import Home from ‘./components/Home.js’; import { browserHistory, IndexRoute, Redirect, Route, Router } from ‘react-router’; import DataActions from ‘./actions/DataActions.js’; class AppInitializer { buildRoutes(data) { return data.pages.map((page, i) => { return ( <Route component={ Home } key={ page.id } path={`/${page.slug}`} /> ); }); } run() { DataActions.getPages((response)=>{ render( <Router history={browserHistory}> <Route path=”/” component={ App } > <IndexRoute component={ Home } /> {this.buildRoutes(response)} </Route> <Redirect from=”*” to=”/” /> </Router> , document.getElementById(‘app’) ); }); } } new AppInitializer().run(); |
Within all pages returned by WordPress API, this method returns the loop and new routes. It also adds the “Home” element along each route.
Page Templates
We can use the page elements to find out which template to use with a specific page. The page slug returned by the API can be used to map templates for different routes.
If there are two-page “slog” home and “about” are present and we need to create a file that will mapping the page slug to the React component paths. We can assume the file name.
views.js and put it in “components” folder:
export default {
‘home’: ‘./components/Home.js’,
‘about’: ‘./components/About.js’
};
Same as About.js should be created to map the “about” slug.
For the update in index.js file, we will make it request the right component dynamically.
import React from ‘react’;
import {render} from ‘react-dom’; import App from ‘./components/App.js’; import Home from ‘./components/Home.js’; import views from ‘./components/views.js’; import { browserHistory, IndexRoute, Redirect, Route, Router } from ‘react-router’; import DataActions from ‘./actions/DataActions.js’; class AppInitializer { buildRoutes(data) { return data.pages.map((page, i) => { const component = views[page.slug]; return ( <Route getComponent={(nextState, cb) => { require.ensure([], (require) => { cb(null, require(component).default); }); }} key={ page.id } path={`/${page.slug}`} /> ); }); } run() { DataActions.getPages((response)=>{ render( <Router history={browserHistory}> <Route path=”/” component={ App } > <IndexRoute component={ Home } /> {this.buildRoutes(response)} </Route> <Redirect from=”*” to=”/” /> </Router> , document.getElementById(‘app’) ); }); } } new AppInitializer().run(); |
views.js has been included in the file and updates have been made in the buildRoutes() method to require the right components.
To collect page-specific data, just call the DataStore.getPageBySlug (slug) method and provide the current page slug:
render() {
let page = DataStore.getPageBySlug(‘about’); return ( <div> <h1>{page.title.rendered}</h1> </div> ); } |
Dynamic Navigation
We will add global navigation to display all WordPress backend page links. Start building the Nav.sj component inside the “Components” folder:
import React from ‘react’;
import { Link } from ‘react-router’; import _ from ‘lodash’; import DataStore from ‘./../stores/DataStore.js’; class Nav extends React.Component { render() { let allPages = DataStore.getAllPages(); allPages = _.sortBy(allPages, [function(page) { return page.menu_order; }]); return ( <header> {allPages.map((page) => { return <Link key={page.id} to={`/${page.slug}`} style={{marginRight: ’10px’}}>{page.title.rendered}</Link> })} </header> ); } } export default Nav; |
DataStore.getAllPages () will fetch all pages from WordPress, moreover which we can sort them by “menu_order” with lodash and looping by them to spit the React Router’s <Link/>.
Include the Nav.js component in App.js to witness the dynamic nav contained on each page:
import React from ‘react’;
import Nav from ‘./Nav.js’; export default class App extends React.Component { constructor(props) { super(props); } render() { return ( <div className=”wrapper”> <Nav /> {this.props.children} </div> ); } } |
That’s all in our behalf and we hope that this article helped you completely.
Get in touch with our support team
If the above guide is not useful and you are still having trouble then don’t panic. Our support team is just one call away to help you. You can contact our professional experts and they will listen to you and will try to provide effective solutions to your problem.
For any help and support Dial our, WordPress Technical Support number +1-888-738-0846 (Toll-Free).